Store Search SDK Embedded
Getting your SDK Key
- To get an API key, book a meeting with us and sign the license agreement. Book a meeting by visiting here.
Store search
Store search can be used to find and order from stores near the user. We also provide multiple options to customize store search to fit your specific use case.
Example images
Grocery:
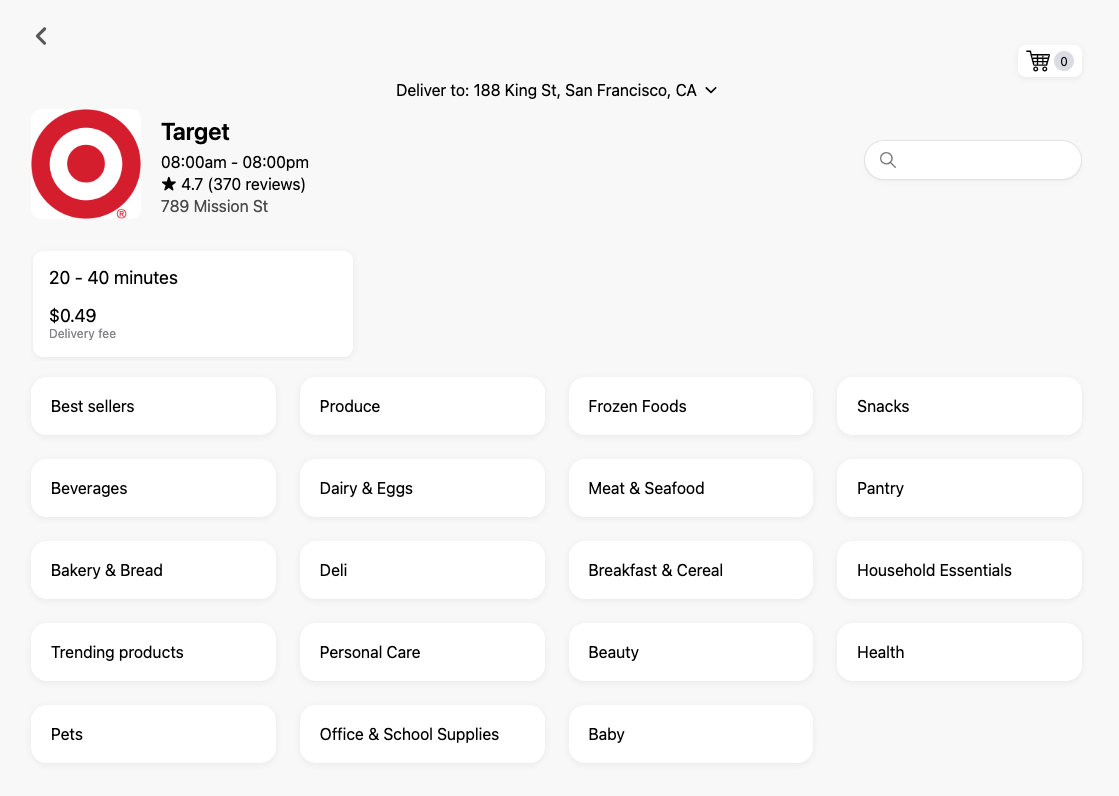
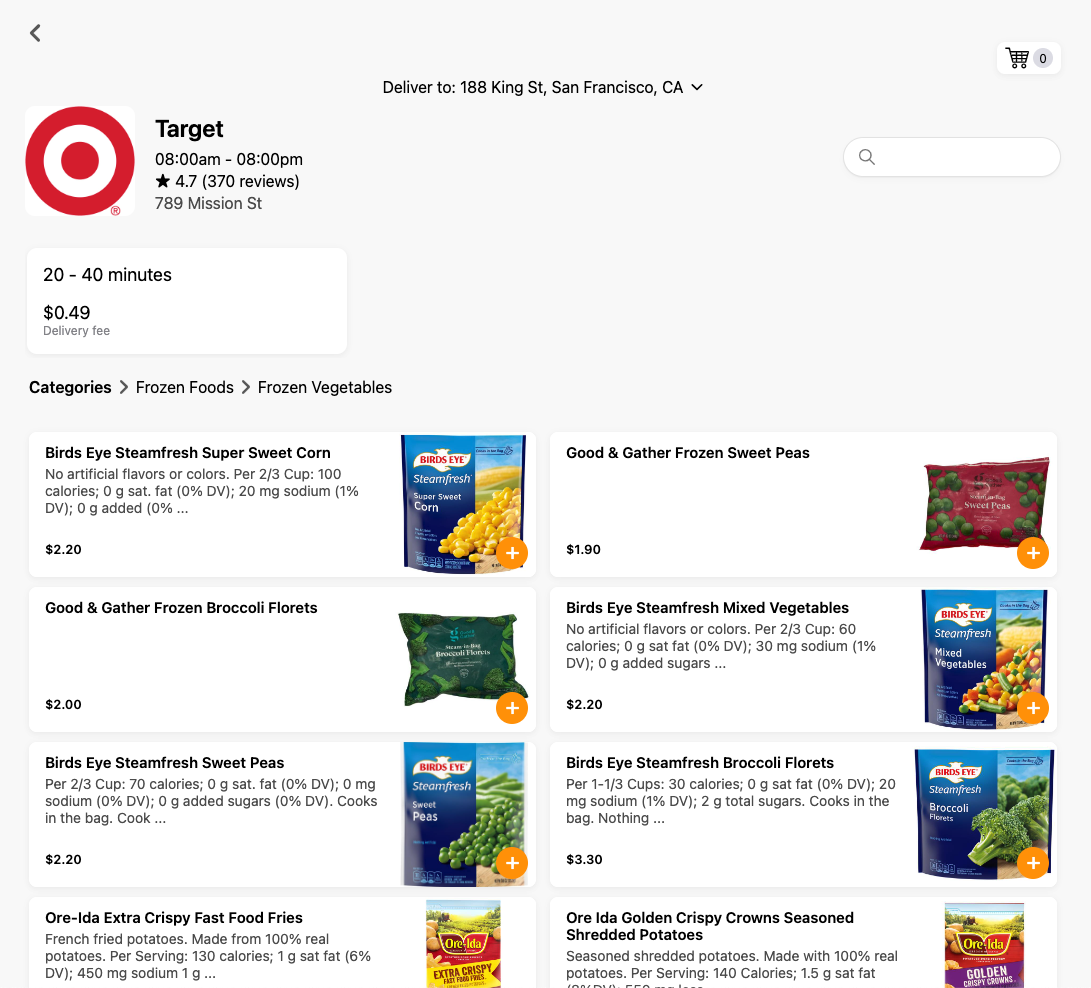
Getting started
Minimum: passing your name
For example:
https://sdk.mealme.ai/store?api=YOUR_NAME
Optional: pass address information
If you don't want us to prompt the user for their location, you can choose to pass address information, ideally all of the following variables. You can get these from the address search SDK.
streetNumber
streetName
city
state
zipcode
country
latitude
longitude
⚠️ NOTE The country is US
for America and CA
for Canada. The state is given in its two-letter abbreviated form, for example: CA
for California, or TX
for Texas.
Example minimal embed:
<iframe
src="https://sdk.mealme.ai/store?api=YOUR_NAME"
allow="geolocation; payment"
title="Mealme Web SDK"
style="border: none;height: 100vh;margin-bottom: 0px;position: relative;z-index: 100;margin-top: 40px;width: 100%;">
</iframe>
Optional customizations
The following optional query parameters are available to adjust the functionality of the SDK:
searchQuery
- a default query for the search, pass for examplesearchQuery=subway
for the initial search to be for subwaystoreType
- Pass eitherrestaurant
orgrocery
, don't include to have results with bothsort
- Will default to this value for sorting stores, accepted values are:relevance
,cheapest
,fastest
,rating
, anddistance
. The default with nothing passed isrelevance
itemSearchQuery
- default search query for items (available forrestaurant
type only)userId
- Use your own identifier for userId if you want an order to be related to a certain user or grouphideSearchbar
passing true will hide the search fieldisPickup
- default value of the pickup toggle, true means it's a pickup order, false means it's a delivery orderisOpen
- default value of the open toggle, true means searching for only open stores, false means to search for all storessearchRadius
- Normally we gradually increase the radius until we find enough stores, pass if you want to override this and just do one specific search radius, is capped at 10 mileshideOpenToggle
- passing true will hide the open togglehideSortDropdown
- passing true will hide the sort dropdownhidePickupToggle
- passing true will hide the pickup toggleuserEmail
- Will prefill the email field in checkout if passeduserName
- Will prefill the name field in checkout if passeduserPhone
- Will prefill the phone field in checkout if passeduserApartmentNumber
- Will prefill the apartment number field in checkout if passedcheckoutNotes
- Will prefill the notes field in checkout if passedtitle
- optional title that displays at the top of the pageerror
- If passed, an error snackbar will appear with this error when the page loadscustomSearchbarText
- Pass to change the placeholder text for the search barstoreIds
- Comma separated store_id's, this will show only these stores in the interface and hide other options, a maximum of is 50 allowed.desiredTime
- If scheduled ordering is available, we will select the timeslot closest to this timestamp. Pass as unix time, for example:1670000000
primaryColor
- Changes the color theme of the SDK from the default orange color. Pass as hex without the #, for example&primaryColor=777777
quotePreference
- The preferred quote for order fulfillment (on the menu). Can bedefault
,first_party
,cheapest
,fastest
,first_available
,cheapest_inventory
, orcheapest_for_store
.disableMenuCheckoutButton
- Passing true hides the checkout button on the menudisableMenuQuote
- Passing true disables the quote fetching on the menu (not recommended)disableCheckoutRedirect
- Passing true prevents the tracking site from being opened in a new browser tab after checkout is completedcombineServiceAndDeliveryFee
- If passing true this will combine both the delivery fee and service fee on checkout, both will be listed under the category "Service fee"combineServiceFeeAndMiscFees
- If passing true this will combine both the service fee and misc fees on checkout, both will be listed under the category "Service fee"disableEmail
- Passingtrue
disables the email field on checkout, meaning it has to come from theuserEmail
query parameter, and is not changeable by the user on checkoutcollectUserAddress
- Passingtrue
collects the user's address on checkout, even if the order is a pickup orderhideQuote
- Passingtrue
disables the quote card on the menu, which shows fees and estimated time to complete the orderhidePromotions
- Passingtrue
disables the promo code section on checkout, can be used if you don't plan on offering promo codesuseSpecialCheckout
- Passingtrue
disables the last step of the checkout, where the payment gateway is. Use this if you want to process payments on your side, see events below for how to get the data, then finalize the order with this endpoint. We don't recommend doing this unless you have a specific reason to.cartTimeout
- Passing will override the default cart timeout of 1 hour. Passed in seconds, for example&cartTimeout=600
would be a 10-minute. When a cart is timed out, it will no longer re-load when returning to the store where it was created.hideStoreRatings
- Passingtrue
hides the store ratings on the store search page.disableSms
- Passingtrue
disables sms communication to the customer after the order is placed
Capturing events
We fire events that you can catch if you want to read the data.
The events fired from the store-search checkout flow are the following:
- Store click
- Checkout success
- Checkout failure
- Special checkout
If you want to capture events while having the SDK in an App, please follow these instructions, instead of the web examples given below.
When user clicks a store
Code for listening to store click event:
<script>
window.onmessage = (event) => {
if (event.data.id === "mealme-store-select") {
// Your code using event.data
}
};
</script>
Example content of event.data:
id: "mealme-store-select"
address: {street_addr: '301 Example Street', city: 'San Francisco', state: 'CA', zipcode: '94121', country: 'US'}
aggregated_rating_count: 500
cuisines: (15) ['By The Slice Pizza', 'Chicken', 'Dinner', 'Healthy', 'Healthy Pizza', 'Lunch', 'Pasta', 'Pickup', 'Pizza', 'Salads', 'Sandwiches', 'Seafood Pizza', 'Subs', 'Takeout', 'Veggie Pizza']
delivery_enabled: true
description: "Sandwiches, Takeout, Pickup, Pizza"
dollar_signs: 3
food_photos: ['https://cdn-img.mealme.ai/example']
is_open: true
local_hours: {
delivery: {Monday: '08:00AM - 09:00PM', Tuesday: '08:00AM - 09:00PM', Wednesday: '08:00AM - 09:00PM', Thursday: '08:00AM - 09:00PM', Friday: '08:00AM - 09:00PM', Saturday: "12:00AM - 08:30AM, 11:00AM - 12:00AM", Sunday: "11:00AM - 09:30PM"}
dine_in: {Monday: '08:00AM - 09:00PM', Tuesday: '08:00AM - 09:00PM', Wednesday: '08:00AM - 09:00PM', Thursday: '08:00AM - 09:00PM', Friday: '08:00AM - 09:00PM', Saturday: "12:00AM - 08:30AM, 11:00AM - 12:00AM", Sunday: "11:00AM - 09:30PM"}
operational: {Monday: '08:00AM - 09:00PM', Tuesday: '08:00AM - 09:00PM', Wednesday: '08:00AM - 09:00PM', Thursday: '08:00AM - 09:00PM', Friday: '08:00AM - 09:00PM', Saturday: "12:00AM - 08:30AM, 11:00AM - 12:00AM", Sunday: "11:00AM - 09:30PM"}
pickup: {Monday: '08:00AM - 09:00PM', Tuesday: '08:00AM - 09:00PM', Wednesday: '08:00AM - 09:00PM', Thursday: '08:00AM - 09:00PM', Friday: '08:00AM - 09:00PM', Saturday: "12:00AM - 08:30AM, 11:00AM - 12:00AM", Sunday: "11:00AM - 09:30PM"}
}
logo_photos: ['https://cdn-img.mealme.ai/example']
miles: 0.2
name: "Example name"
offers_first_party_delivery: false
offers_third_party_delivery: true
phone_number: 12345678901
pickup_enabled: true
quote_ids: ['example']
store_photos: ['https://cdn-img.mealme.ai/example']
type: "restaurant"
weighted_rating_value: 5
_id: "1234567-1234-1234-1234-aaa1234567"
Checkout success event
Code to capture success event
window.onmessage = (event) => {
if (event.data.id === "mealme-checkout-success") {
// Your code using event.data
}
};
Example data for success event:
{
success: true,
orderId: "123",
items: [
{
product_id: "gAAA",
item_name: "NESCAFÉ Dark Roast Instant Coffee",
image: "https://cdn-img.mealme.ai/...",
description: "",
category: "Coffee",
price: 1299,
formatted_price: "$12.99",
upc_codes: [],
unit_size: 10.5,
unit_of_measurement: "oz",
should_fetch_customizations: true,
},
{
product_id: "gAAAA",
item_name: "Lactaid Whole Milk",
image: "https://cdn-img.mealme.ai/...",
description: "",
category: "Milk",
price: 579,
formatted_price: "$5.79",
upc_codes: [],
unit_size: 0.5,
unit_of_measurement: "gal",
should_fetch_customizations: true,
},
],
trackingLink: "https://tracking.mealme.ai/tracking?tracking_id=123",
};
Checkout fail event
Code to capture fail event
window.onmessage = (event) => {
if (event.data.id === "mealme-checkout-fail") {
// Your code using event.data
}
};
Example data for success event:
{
success: false,
message: "Something went wrong",
}
Special checkout events
Code to capture events
window.onmessage = (event) => {
if (event.data.id === "mealme-unfinished-order-created") {
// event.data.order_id contains the order id you then finalize with the API
}
if (event.data.id === "mealme-special-checkout-click") {
// Open your payment gateway after this
}
};
Updated about 2 months ago