Cart Search SDK Embedded
Getting your SDK Key
- To get an API key, book a meeting with us and sign the license agreement. Book a meeting by visiting here
Cart search
Example images
Cart search:
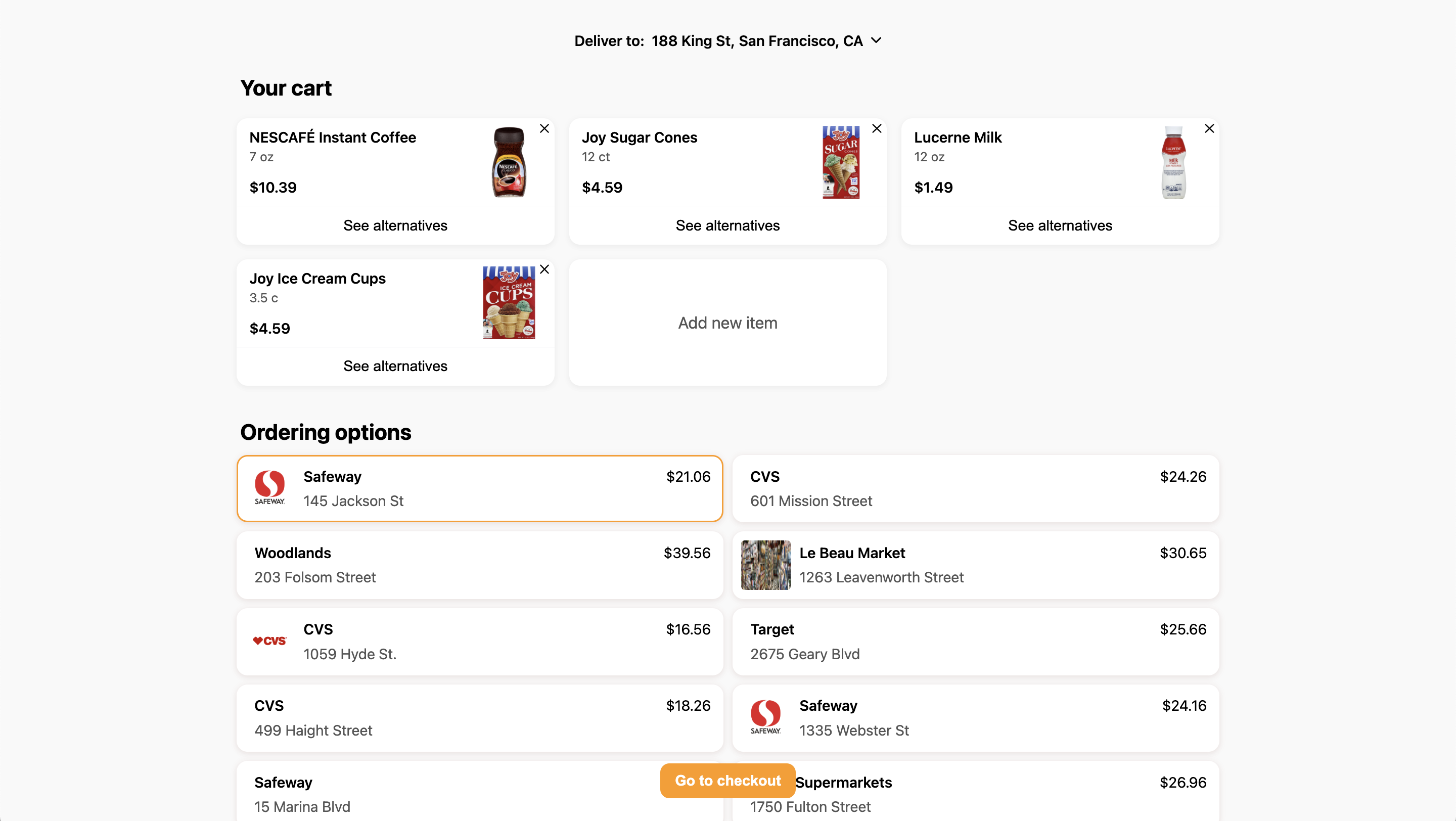
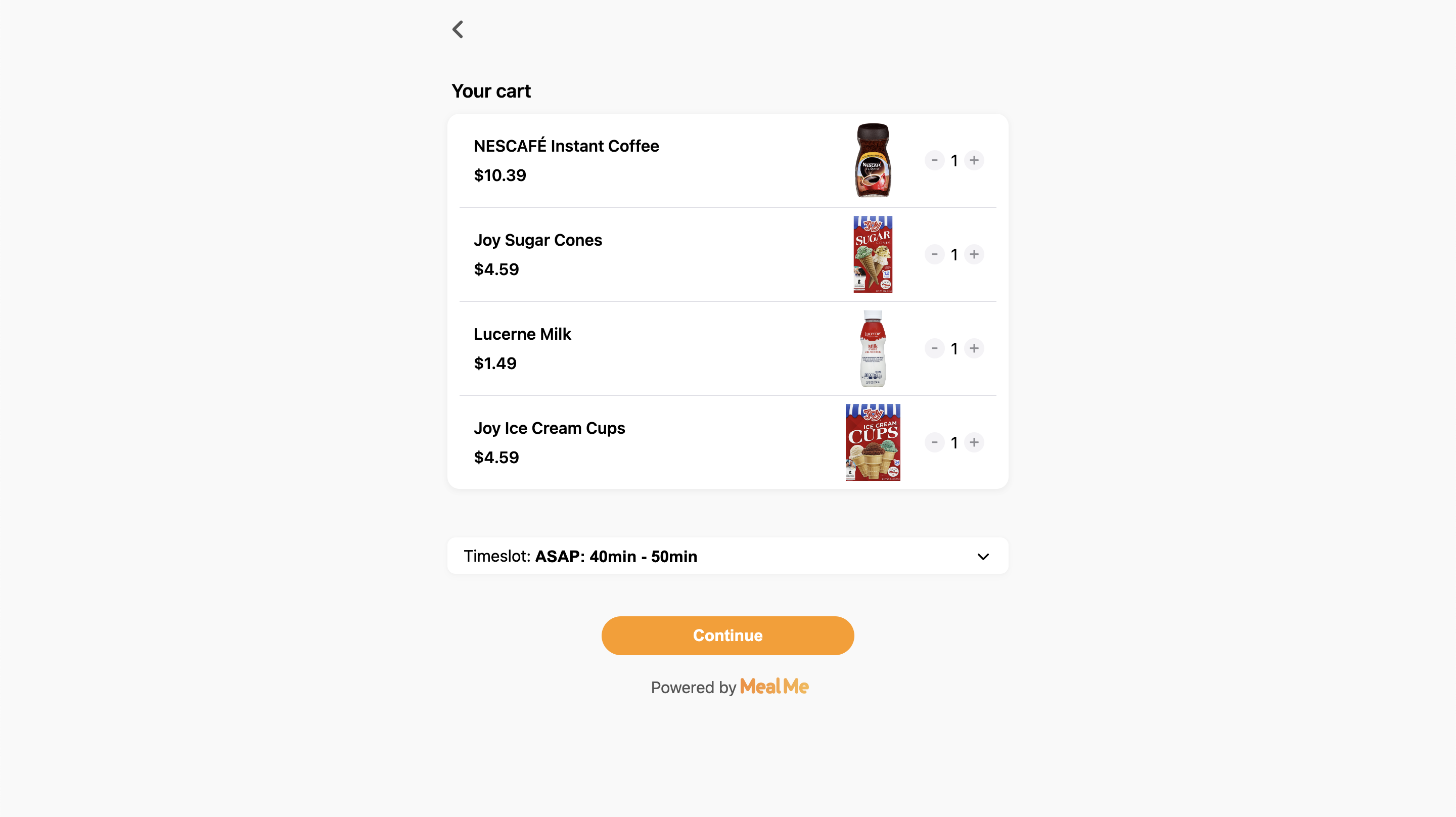
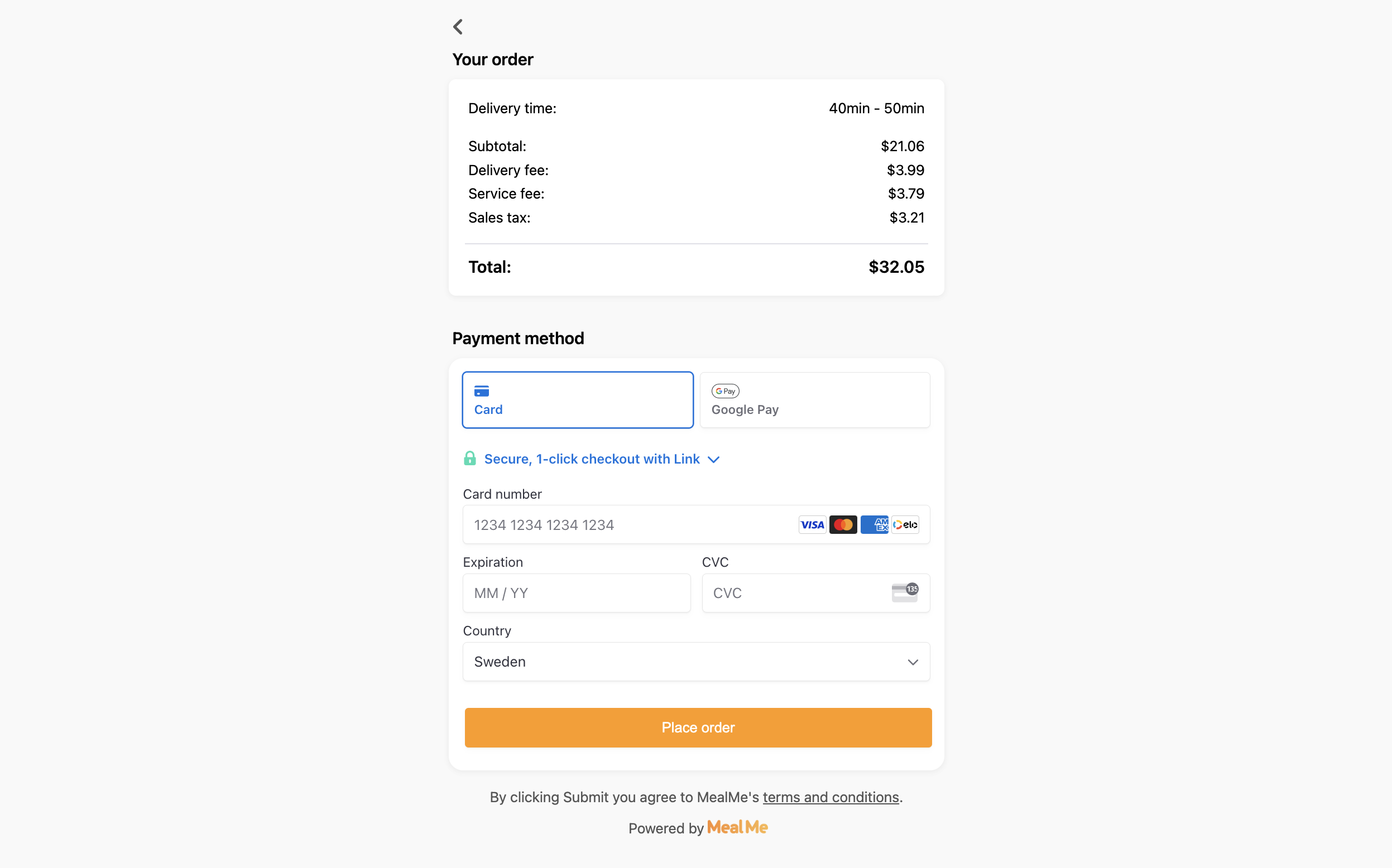
Minimum: passing name and query
At a minimum, you need to pass your company name and the query. For extra options see "Additional optional query parameters" further down on this page.
Query
Query is simply a list of items and the quantity you want, followed by the quantity wanted of that item.
For example:
https://sdk.mealme.ai/cart-search?api=YOUR_NAME&query="Eggs":1,"Milk":1
⚠️ Note: YOUR_NAME will be the name of your company, for sandbox orders use YOUR_NAME-sandbox
Passing location
If you already have the user's location you can pass that information, this way they would not have to enter their location
Example with location parameters:
Additional optional query parameters
primaryColor
- Changes the color theme of the SDK from the default orange color. Pass as hex without the #, for example&primaryColor=777777
isPickup
- default value of the pickup toggle, true means it's a pickup order, false means it will be a delivery orderisOpen
- default value of the open toggle, true means searching for only open stores, false means to search for all storeshidePickupToggle
- passing true will hide the pickup togglehideOpenToggle
- passing true will hide the open toggletitle
- If passed, it will show a title above the cart search user interfacelogoUrl
- If passed, a logo will be added, in the top left by defaultlogoHeight
- If passed it will change the width of the logo, the default value is 30logoAlignment
- Can be used to change the alignment of the logo, accepted values are left, center, and rightsearchRadius
- Normally we gradually increase the radius until we find enough stores, pass if you want to override this and just do one specific search radius, is capped at 10 milessort
- Will default to this value for sorting stores, accepted values are:relevance
,cheapest
,fastest
,rating
, anddistance
. The default with nothing passed isrelevance
fullCartsOnly
- only show full carts, it's defaulted to falseuserId
- Use your own identifier for userId if you want an order to be related to a certain user or groupuserEmail
- Will prefill the email field in checkout if passeduserName
- Will prefill the name field in checkout if passeduserPhone
- Will prefill the phone field in checkout if passeduserApartmentNumber
- Will prefill the apartment number field in checkout if passedcheckoutNotes
- Will prefill the notes field in checkout if passedstoreType
- Passrestaurant
for restaurant items, passgrocery
for grocery items, or leave empty or exclude to search for bothpinCheckoutButton
- If passingtrue
this will pin the checkout button to the bottom element instead of having a floating buttondisableMenuCheckoutButton
- Passing true hides the checkout button on the menudisableMenuQuote
- Passing true disables the quote fetching on the menu (not recommended)combineServiceAndDeliveryFee
- If passingtrue
this will combine both the delivery fee and service fee on checkout, both will be listed under the category "Service fee"combineServiceFeeAndMiscFees
- If passing true this will combine both the service fee and misc fees on checkout, both will be listed under the category "Service fee"disableEmail
- Passingtrue
disables the email field on checkout, meaning it has to come from theuserEmail
query parameter, and is not changeable by the user on checkoutcollectUserAddress
- Passingtrue
collects the user's address on checkout, even if the order is a pickup orderhideQuote
- Passingtrue
disables the quote card on the menu, which shows fees and estimated time to complete the orderhidePromotions
- Passingtrue
disables the promo code section on checkout, can be used if you don't plan on offering promo codesuseSpecialCheckout
- Passingtrue
disables the last step of the checkout, where the payment gateway is. Use this if you want to process payments on your side, see events below for how to get the data, then finalize the order with this endpoint. We don't recommend doing this unless you have a specific reason to.cartTimeout
- Passing will override the default cart timeout of 1 hour. Passed in seconds, for example&cartTimeout=600
would be a 10-minute. When a cart is timed out, it will no longer re-load when returning to the store where it was created.exhaustiveSearch
- If passingtrue
you will get better result at the cost of slightly longer loading time, we recommend using this for most implementationsitemTimeout
- How much time, in milliseconds, that is spent on each item, is ignored it exhaustive search is used.itemsPerSearchTerm
- How many maximum items are returned per item in the passed cart query. It can be changed to improve loading time, but not recommended in most cases.disableSms
- Passingtrue
disables sms communication to the customer after the order is placed
Example embed
⚠️ NOTE The below is a html example, for mobile applications (React Native etc.), see page: https://docs.mealme.ai/docs/sdk-mobile-implementation
<iframe
src='https://sdk.mealme.ai/cart-search?api=YOUR_NAME&query="Eggs":1,"Milk":1'
allow="geolocation; payment"
title="Mealme Web SDK"
style="border: none;height: 100vh;margin-bottom: 0px;position: relative;z-index: 100;margin-top: 40px;width: 100%;">
</iframe>
Capturing events
We fire events that you can catch if you want to read the data.
The events fired from the cart-search checkout flow are the following:
- Checkout success
- Checkout failure
If you want to capture events while having the SDK in an App, please follow these instructions, instead of the web examples given below.
Success event
Code to capture success event
window.onmessage = (event) => {
if (event.data.id === "mealme-checkout-success") {
// Your code using event.data
}
};
Example data for success event:
{
success: true,
orderId: "123",
items: [
{
product_id: "gAAA",
item_name: "NESCAFÉ Dark Roast Instant Coffee",
image: "https://cdn-img.mealme.ai/...",
description: "",
category: "Coffee",
price: 1299,
formatted_price: "$12.99",
upc_codes: [],
unit_size: 10.5,
unit_of_measurement: "oz",
should_fetch_customizations: true,
},
{
product_id: "gAAAA",
item_name: "Lactaid Whole Milk",
image: "https://cdn-img.mealme.ai/...",
description: "",
category: "Milk",
price: 579,
formatted_price: "$5.79",
upc_codes: [],
unit_size: 0.5,
unit_of_measurement: "gal",
should_fetch_customizations: true,
},
],
trackingLink: "https://tracking.mealme.ai/tracking?tracking_id=123",
};
Fail event
Code to capture fail event
window.onmessage = (event) => {
if (event.data.id === "mealme-checkout-fail") {
// Your code using event.data
}
};
Example data for fail event:
{
success: false,
message: "Something went wrong",
}
Updated about 2 months ago