Address Search SDK Embedded
How to embed the address search SDK
Getting your SDK Key
- To get an API key, book a meeting with us and sign the license agreement. Book a meeting by visiting here.
Build-in features
- Use Current Location: Getting longitude/latitude from the browser and using reverse geocode to get an accurate address
- Autocomplete address suggestions as the user types
- Location-based autocomplete suggestion using IP-address to ensure suggestions are relevant
Embedded Implementation
Example implementation
See "Full embedded example" below for this example.
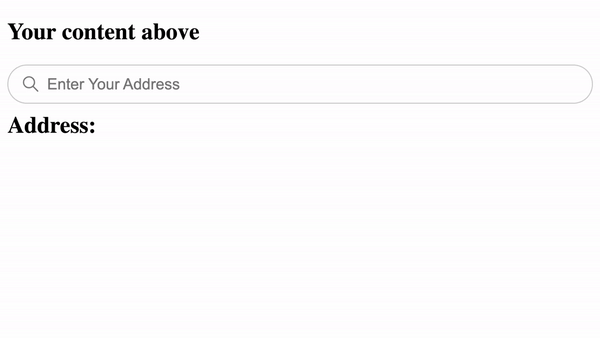
1. Embedding
Put the following element somewhere in the body element of your HTML. Replace YOUR-NAME
with your name.
⚠️ NOTE The below is a html example, for mobile applications (React Native etc.), see page: https://docs.mealme.ai/docs/sdk-mobile-implementation
<iframe
src="https://sdk.mealme.ai/address?api=YOUR-NAME"
allow="geolocation"
title="MealMe Address Search Web SDK"
style="border: none; width: 100%; height: 350px; margin-bottom: -325px; position: relative; z-index: 100;">
</iframe>
Style can be changed, but we recommend keeping it the way it is to get the expected behaviour.
2. Listening to events
To know when an address is chosen, you can add an event listener and check the event with the following code. You can add this anywhere in the body of your HTML.
<script>
window.onmessage = (event) => {
if (event.data.id === "mealme-address-chosen") {
// Your code using event.data
}
};
</script>
Example of how data from the event can look (event.data):
{
id: "mealme-address-chosen",
address: {
street_num: '100',
street_name: 'Centre St',
city: 'New York',
state: 'NY',
zipcode: '10013',
},
latitude: 40.71433469999999
longitude: -74.0015312
name: "100 Centre St, New York, NY 10013, US"
}
Full embedded example
⚠️ NOTE the area below the search bar is not interactable in this example, if you need it to be, see "Interactable below full example"
If you want a complete example, here is an HTML file you can use to test the complete implementation. Copy this code and place in a new file example.html
and replace YOUR-NAME
with your name.
<html>
<body>
<script>
window.onmessage = (event) => {
if (event.data.id === "mealme-address-chosen") {
console.log(event.data);
document.getElementById("address").innerHTML = `Address: ${event.data.name}`;
}
};
</script>
<h2>Your content above</h2>
<iframe
src="https://sdk.mealme.ai/address?api=YOUR-NAME"
allow="geolocation"
title="MealMe Address Search Web SDK"
style="border: none; width: 100%; height: 350px; margin-bottom: -325px; position: relative; z-index: 100;">
</iframe>
<h2 id="address">Address:</h2>
</body>
</html>
Interactable below embedded example
If you want a complete example, here is an HTML file you can use to test the complete implementation. Copy this code and place in a new file example.html
and replace YOUR-NAME
with your name.
<html>
<body>
<script>
window.onmessage = (event) => {
console.log(event);
if (event.data.id === "mealme-address-field-focus") {
document.getElementById("address-iframe").style.height = "350px";
document.getElementById("address-iframe").style.marginBottom = "-308px";
} else if (event.data.id === "mealme-address-field-unfocus") {
document.getElementById("address-iframe").style.height = "42px";
document.getElementById("address-iframe").style.marginBottom = "0px";
} else if (event.data.id === "mealme-address-chosen") {
console.log(event.data);
document.getElementById("address").innerHTML = `Address: ${event.data.name}`;
}
};
</script>
<h2>Your content above</h2>
<iframe
id="address-iframe"
src="https://sdk.mealme.ai/address?api=YOUR-NAME"
allow="geolocation"
title="Mealme Web SDK"
style="border: none; width: 100%; height: 42px; margin-bottom: 0px; position: relative; z-index: 100;"
></iframe>
<h2 id="address">Address:</h2>
</body>
</html>
Updated about 1 month ago