Product Details SDK Embedded
Getting your SDK Key
To get an API key, book a meeting with us and sign the license agreement. Book a meeting by visiting here.
Examples
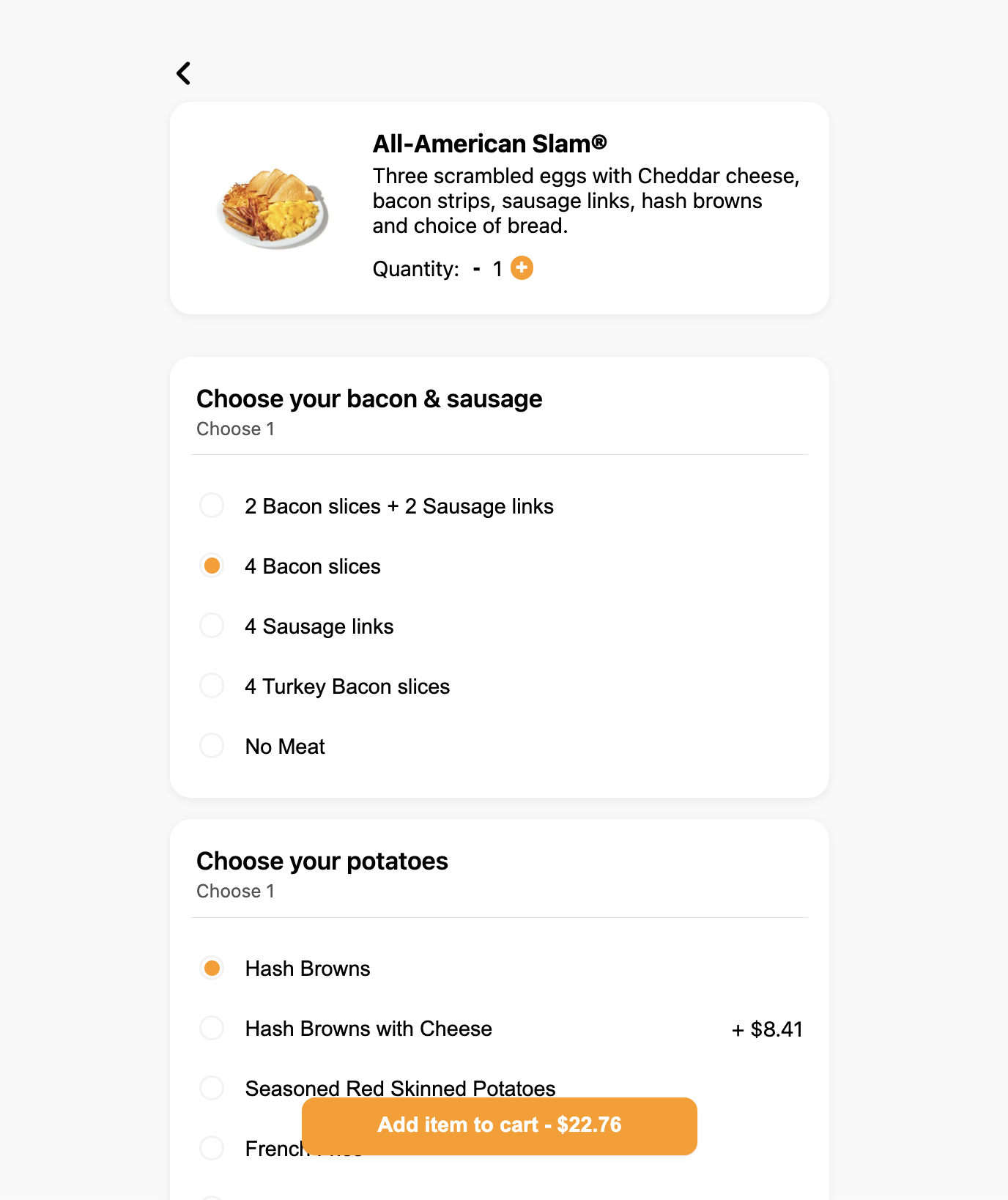
Required parameters: name, productId, and isPickup
Your name is passed with the api query parameter. This will be the name of your company.
The productId will be the id of the product you want to customize.
isPickup
- defines whether the order will be pickup or delivery, please pass isPickup=true
if the order will be a pickup order. If nothing is passed, a delivery order is assumed
For example:
https://sdk.mealme.ai/product-details?api=YOUR_NAME&productId=12345
Example embed:
⚠️ NOTE The below is a html example, for mobile applications (React Native etc.), see page: https://docs.mealme.ai/docs/sdk-mobile-implementation
<iframe
src="https://sdk.mealme.ai/product-details?api=YOUR_NAME&productId=12345"
allow="geolocation"
title="Mealme Web SDK"
style="border: none;height: 100vh;margin-bottom: 0px;position: relative;z-index: 100;margin-top: 40px;width: 100%;">
</iframe>
Optional: pass address information
If you don't have your own way of getting them. To pass the address, please pass all the following variables as query parameters:
streetNumber
streetName
city
state
zipcode
country
latitude
longitude
⚠️ NOTE The country is US
for America and CA
for Canada. The state is given in its two-letter abbreviated form, for example: CA
for California, or TX
for Texas.
Optional parameters
The following optional query parameters are available to adjust the functionality of the SDK:
primaryColor
- will change the color theme of the product details SDK. Pass as hex without the #, for example&primaryColor=777777
hideHeader
- will hide the header with product information if true is passedhideBackButton
- will hide the back button if true is passedisNewItem
- true by default, if passing false, the CTA button will say "Save item options" instead of "Add item to cart"includeQuote
- checks the availability of the store if passed astrue
(&includeQuote=true
), if passed, also includeisPickup
to define whether to check pickup or delivery availabilityisPickup
- ifincludeQuote=true
, include this to indicate whether to check pickup (&isPickup=true
) or delivery (&isPickup=false
) availability,
To check the delivery availability, you also have to pass all the following variables as query parameters:
For example:streetNumber streetName city state zipcode country latitude longitude
https://sdk.mealme.ai/product-details?api=YOUR_NAME&includeQuote=true&isPickup=false&productId=x&latitude=x&longitude=x&streetNumber=x&streetName=x&city=x&state=x&country=x&zipcode=x
Capturing events
We fire events that you can catch if you want to read the data.
The events fired from Product Details SDK are the following:
- Customizations success
- Item not available
- Customization exit
If you want to capture events while having the SDK in an App, please follow these instructions, instead of the web examples below.
NOTE: if you want to use multiple of the events, place the if statements in the same onmessage
listener, otherwise redefining the onmessage
will override the existing one.
Success event
Code to capture the success event
window.onmessage = (event) => {
if (event.data.id === "mealme-customizations-complete") {
// Your code using event.data
}
};
Example event.data for success event, this object can be passed as an item to the order API:
NOTE: the product_id in the event can be different from the one passed in the query parameter. If the passed id is no longer valid, a newer valid id can be returned instead.
{
"notes": "",
"product_id": "gAAAAAAAAAAAvcAsFofXR7XlzNv5B6-g-keD0Ml6ZOFGjTcoMQPE2h0H3sEEwRwzs-DAVDzr1NugDYUHyUe2gAxZH6aL_WienyP4TBaoBvefXAX-7PnRSfDciPkCb-FdFdP-sSrBWbpvlmiok3vlBB34ErwH-BSvPmeGZu9ZaHlGwNDeuYoQYheaP-HE46VzC6JH2Rl1jryNcElIKbLufw-412IzisK9-kUBc1ldR4Zm76Y8iQ9emp7nuCWyjZVej2IMmvgdf6q8JnXLBouY3XUDwE2ED2cvzzcHd0PcAwtbYlF1XqLYhkBxur3XRbBVr_7B5UuKjy9a2ies_nebrjc1-7KR-H04RHjf3oul3rNULTX8fxvd5glvRRLcGCwLHIYxxlMK8IBU5RBvFZ8KtGefZanKfExYECxlE-YmPUyWrnnnpwB1HS3eBz1KmUKo8o_M65V4FybL0umisAjM6LqpgEVJtVsUmXNze31EKyZozufuGubjQLUkmd2tS6D5FoyYMNZ5DpZ5F9_wKa_UbpESIcRoR9-9BCflffPyci2OOhLeRXtwhWoArcERWHcwyWJVbt0TsfSJwJx8vwg-LyMbpr4Jc-1SPESC6pkD8_Wiy93KNgVvIWk_bdTcg3fQsQW8OVYLWIMDYt4BqAfaVWWAIz4vHKadBr-H8bRLalU5VCytRgheawVhzE4i6Bt0fVdvWFWiO6MVaea2ihdL9sZxtBGR5QzAMT2XsAtnNqkulmHiXCTjW7q07A-dhECiR3r_bWLGddGxhLBQmiflSLOYvIBwaadZUjWbrDzqUONH9q1ssP5jiJRYtdcfYbFIgYYsnMpJ3FDQNCCujJ_fMKQmubz4lkFE_w==",
"selected_options": [
{
"name": "No Tomatoes",
"option_id": "gAAAAAAAAAAAvcAsFofXR7XlzNv5B6-g-uWBO4rWaV1NifPmsOG_vz8oJH3OKddE7KAx9Uz1eny6cEj62MM08GKLC3wMOiEql4VlbwRCt8h0G3YmRY0GKLZU0mz_4rytIkHWZgWv41ijuZbCZdOwiDpExJpwTcow2UYXrKoJ1-OXW98K1T4w8X60vMqRShlJHlJnJMQD6s7VqeJW5ywQvzn6E5AQkhMwwCLfuGHztOcxIRbFmI04HdRN7GeAyiaXKMqfYwYjIlQeMNvEBZnV1G9dKq-J7Q7XaqiaQcqkqpNJMmKlA77yyI1mJ_RoILIIVITy9O1hTB9ng3sCNLZoPM2hEmVzWKBaOEeJ60srJUkQbwDa_1jQ4DL7J05gt7IqrZPoH35UQU4wwVO9ZpiW8WMvbz71_7Yddv9ueUHEPLTaIi2ICDorFeU3ILZvwhJ0Ta2h1mKXif8pcRcD8jmzkapKdYG1vW13OQXUK3hV2H1URlCpTCGZBMfyknABC1eGW1Be-5i9SUUMH1V5aMPzgCOvJb0KaPe_5Q==",
"quantity": 1
},
{
"name": "Salad Wrap",
"option_id": "gAAAAAAAAAAAvcAsFofXR7XlzNv5B6-g-qmF38dqlZmLgX3MkAD1hqrwNgoW8I9pdJUScxuT9e5ELdIc00Ui-QW8wdKH3-fHF_U2v4O3E6LAnyMeHBWyO02z26SZxvOzqAo_ca-wbe2iPieVPH4qpiSY-JgL-pja2CTGj06sY3pak5jujhhfFlaFvHMSEArXokfeh1t0XI6jGIUbHBbvhaPMdnf4YUojPWX_XcQq70ptW3ZC56r-h8_FYDPkv_xtPjuFFWKNdPO5iDMraIL5Onjc3UG9ZyVq8ZMkLnBkz0hdVKqC0vilF8RLxBreYRtnFe7X3o7uTwRKiorz4sOLebMgo9PVodku7a_qXS-OEKXTeo1TcP80yNVeCwVScHAfPH4PhdvUW35LdxUDFnRxXqhLRx_6isF9pcHeK43K1e_ZmyhhUULdq0ZwbaS_5b_TVIA3tQVO-NVTNEOuxL5uN363djuLraSeNFVqlmLRUU2iwLsPN4H-fbH4ULvGyMTZjvPMIYUboOjIW7AEPebOUJ5akBFfoq9z2c8elYsGP2Gvz6Ul_SEvrWnq4z7bcTIIB_wWcY21Yd7b",
"quantity": 1
},
{
"name": "American Cheese",
"option_id": "gAAAAAAAAAAAvcAsFofXR7XlzNv5B6-g-iuRM7UZj0MMaz8VoBszM_9EnZxs2HFxiZ4TtqsSc66eazYNbgdMlbAYSqN75q_35W9uFqGSj8PNPKTPhNGjJe3r1N0xfKK_zAlRD1hglMewkjGrANigOmUkLY-tdpkFYsnS4QTPOWqWSWEbAmvy8hDjJqtYRciLcE5kJCG2FqcjAvlJQTVJk0roxtCskvCILmqMcg8tjZ1NkbhnItnKpKUL_X1ErPi7KIs330_hyTzyD8S5uO-m-sRF87BxnP8Icksm-Pk1-cXt0FK_QXCNs5AIhM70xZD7rPv9hXlRHsPhPff8F7mUusot3tdctSY3tM8taVuS0og5yyT5ng1aHz4JOJzT7VBtRJt_mmWajeaUgfEshHAVHLbUmbK1q6irp6kQxWREjh-Ai7h6emylN622c8R9Ln8P1h5GB11fsr3ldgk0G6PjEB2bbZ4O6uK-p666SgsOdyqHZbhUj-2myjUmsjDBolhEa7vTQ5F5AnpChUBjA1fftH8XlpOqXlBgqtcSb2elTqaD3S9l6vNLWGdQPOsrlxYArNnADjF3C2fXuub6OauaJCHZ8JY02dYEaA==",
"quantity": 1
},
{
"name": "Honey Mustard",
"option_id": "gAAAAAAAAAAAvcAsFofXR7XlzNv5B6-g-nDRBsGtnwtwXjvEXB4TwN0NlwQnt92HjdUDfq0DYwtMDutLEuGSKZ03JeR-MhuqbizmBw1-D7j10LNmnVe1o0xvLP7-iEyVrIbar4p3ixWWTYcGLwXUVMd79b8mjTZ3jHjNGUu6ppbPwYvQjO5rjHjhWJceSE5xSMEJ64k4TY6MFeD_NLwldG2Z1JkOATzIZ_5To6qTbACla-fS9LoyXdToIbpe7MMd7UZ_qfNJ6G_K9GqFg860xj_oUhFr5T5sZtKvzDQaAYLytVe_2xRCBhNINe9c51ndhwkVvqoduuun04T_NPR4YZMQSbvMbWElHWtIisJ1T2BOStLHZP7DTnb1wQbtcMRI2S_ZC-BVdh6wGOg_v38-x9hS5kFsUp1UOTnw-zA7Ia8Qb3s7sniA1U3ztQvcYEPxI1Y1lumwfSLjRgm9TvTjZCG_KwFHcwIwVNaSS6qsVkCCkphOUVhS8tEv6lB9uHEd11C3QamYRQNaTyIeX7ByAP3lK68yaTSXLzZht_amOO8aF3eyIAfrKrKZdpoOiUox5OJVS4gKySCeOrbGNtf9NfS7GgYXa4GRvg==",
"quantity": 1
},
],
"totalCents": 1078,
"totalFormatted": "$10.78"
}
Not available event
Code to capture the not available event, will be fired if the item or store is not available, for example when using includeQuote=true
and the store is not available
window.onmessage = (event) => {
if (event.data.id === "mealme-item-not-available") {
// Your code here
}
};
Exit event
Code to capture the exit event, will be fired if the user clicks the back button
window.onmessage = (event) => {
if (event.data.id === "mealme-customizations-exit") {
// Your code to exit the SDK
}
};
Updated 20 days ago